The classic card game
Concept
This project was made to improve my C# coding abilities in one week. My other projects rely usually on Native system but I wanted to recreate a similar game that I made in JS. The goal is to have minimal graphics and to have good code with smooth tweening animations.
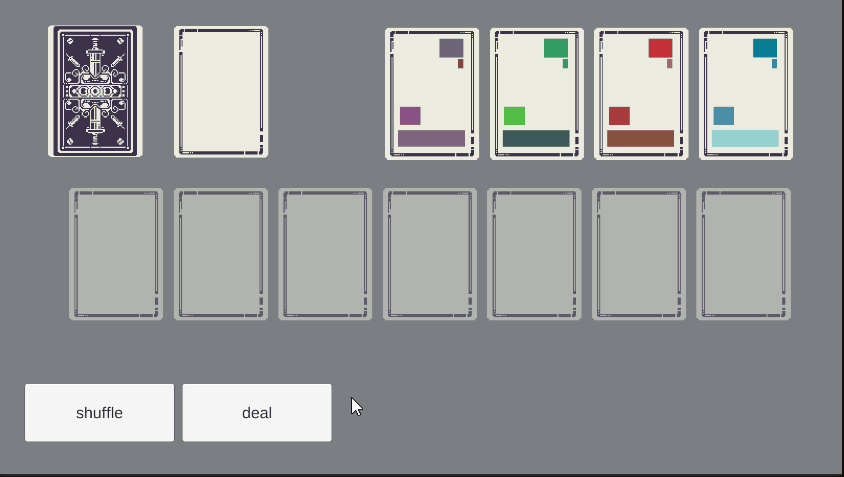
_______________________________________________________________________
public void ShuffleDeck()
{
for (int i = deck.Count - 1; i > 0; i--)
{
int j = Random.Range(0, i + 1);
Card temp = deck[i];
deck[i] = deck[j];
deck[j] = temp;
}
}
public void DealInitGame()
{
for (int i = 0; i < 28; i++)
{
Card cardTemp = deck[0];
deck.Remove(cardTemp);
tableau_deck.Add(cardTemp);
}
int deckIndex = 0;
for (int i = 0; i < 7; i++)
{
for (int j = 0; j <= i; j++)
{
Card card = tableau_deck[deckIndex];
deckIndex++;
card.gameObject.transform.
SetParent(tableauTransforms[i]);
card.gameObject.transform.
localPosition = new Vector3(0, -j * 0.5f,0f);
card.gameObject.
GetComponent().sortingOrder = j;
card.SetIsOnTableau(true);
if (j == i)
{
card.FlipCard();
card.SetTopOfStack(true);
}
}
}
}
_______________________________________________________________________
Deck scripts
Those scripts describe the functions to manage the deck with the shuffle and the first deal.
The shuffle is made using a temp list to assign random positions between two lists.
THe first deal is made by creating each column of the set as lists and then assigning X cards into it.
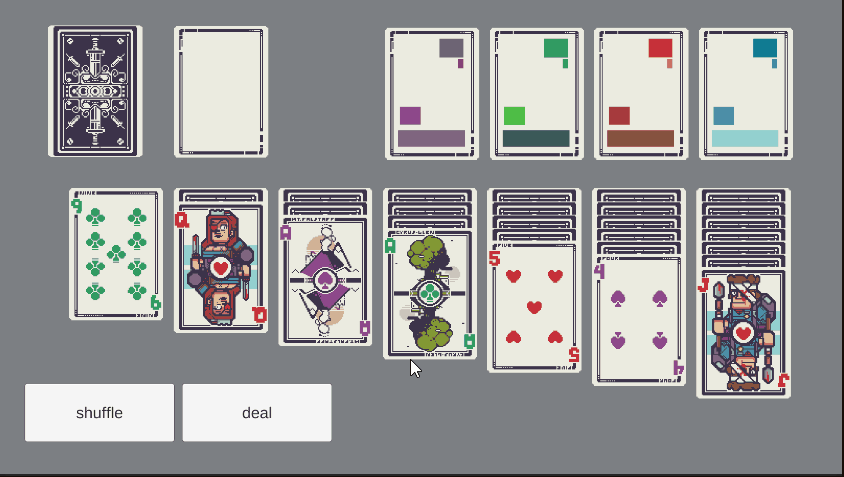
Animations
Animations are made using DOTween function like this one to create a shake when the card is selected and moved:
tween = this.gameObject.transform.DOShakeRotation(1f).SetEase(Ease.Linear).SetLoops(-1);
Tweener sequences
Sequences allow to stack animations with some methods between them in the right order with delegates. The following one will rotate the sprite of the card and when the sprite is invisible to the player (90°), the sprite will swap and then finish the rotation. This is used to "return" a card and changing the backsprite with the face sprite. Another solution would have been to create a 3D object with two faces but the control in the script can be finetuned more easely.
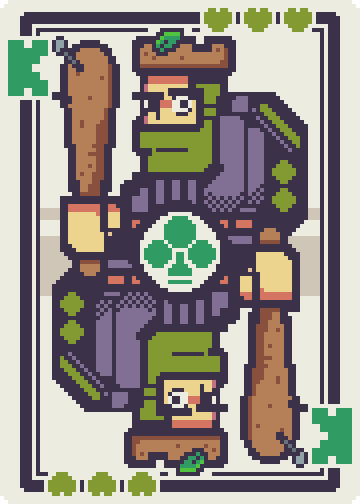
Conclusion
This project was really fun thanks to the massive use of delegates, tweeners and boolean controls. Animations were fun to do because it was
only controlled by the code. We can improve this by creating animated card frames to add more life to the tableaux.
Managing 2d sprites order only by scripts is tricky and it is easy to have cards hidden behind others during tests.
This game could be improve by addind particles effects and by creating a scoring system.