Kuru Kuru Kururin clone template
inspired by a GBA game
UNITY HELIX 2D
This game is inspired by an old classic arcade game from 2001 on GBA published by Nintendo.
The goal of the game is to reach the end of each level within the time limit avoiding obstacles.
The game is composed of a lifebar, a timer with record system, a checkpoint system and animations.
This project aims to recreate the main mechanics of the original game, adding a roster of obstacles,
creating open areas with secondary objectives and a system of bonus/malus to collect to influence several variables in the game.
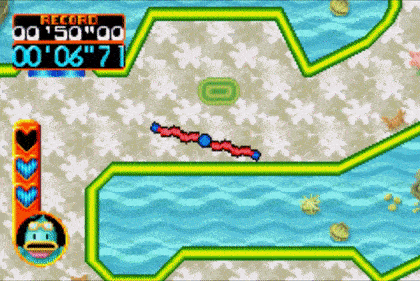
This project was perfect to test the Unity native events when we have a small scene with only one character.
The logic is that everything that happened to the player is a event trigger by all other objects.
This allows to avoid to set references in the player and let each object catch the player reference at the init.
The following code snippet shows how the event class is used to let each bonus to be configured in the editor
without the need to declare each event like with the C# event system. The Unity event system is enought for this small game. .
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Events;
public class BonusMalus : MonoBehaviour
{
public UnityEvent myEvent;
private void OnTriggerEnter2D(Collider2D other)
{
if (other.CompareTag("Player"))
{
myEvent?.Invoke();
Debug.Log(name + " is collected");
Destroy(gameObject, 0.1f);
}
}
private void OnCollisionEnter2D(Collision2D collision)
{
if (collision.gameObject.CompareTag("Player"))
{
myEvent?.Invoke();
Debug.Log(name + " is touched");
//Destroy(gameObject, 0.1f);
}
}
Below we can see how the editor is used for each bonus prefab to choose a method like we would do with the Unity button script.
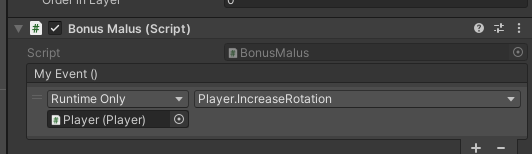
This code is a portion of the methods on the Player C# script. It is easier in our case to create a method to increase and another one to decrease each variable than create a method where we have to pass a sign parameter. Also each variation is then executed by a tweener.
[...]}
public void IncreaseSpeed()
{
moveSpeed *= speedModifier;
}
public void DecreaseSpeed()
{
moveSpeed /= speedModifier;
}
public void IncreaseRotation()
{
rotationSpeed *= rotationModifier;
}
public void DecreaseRotation()
{
rotationSpeed /= rotationModifier;
}
public void ChangeRotationAxe()
{
rotationSpeed = -rotationSpeed;
}
public void IncreaseWingSize()
{
ailes.transform.GetChild(0).
DOScaleX(ailes.transform.GetChild(0).transform.localScale.y + 1, 0.3f);
}
public void DecreaseWingSize()
{
ailes.transform.GetChild(0).
DOScaleX(ailes.transform.GetChild(0).transform.localScale.y - 1, 0.3f);
}[...]
The next code snippet is simple and allows us to manage the checkpoints during a level by passing an array in
the PlayerPrefs. The higher index is the higher checkpoint, the array is fed at the start of the level by assigning an index to a
bunch of Transform representing the chechpoint.
The designer must be careful by creating the level because the order of children in the Checkpoint gameobject must be the same
as the expected level. With a more complex code we could have create a less strict leve design process.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Checkpoint : MonoBehaviour
{
public int index;
private void OnTriggerEnter2D(Collider2D collision)
{
if (collision.CompareTag("Player"))
{
if(GameManager.instance.checkpointIndex < index)
{
GameManager.instance.checkpointIndex = index;
GameManager.instance.checkpointKnow = this.gameObject.transform.position;
Debug.Log("index is now" + index);
}
}
}
}
The last point is that the walls and the floor are made with a Shape sprite Path that allows to create the level with only two object
and adding/removing the nodes from the path. The creation is way faster than placing modular elements for the walls. But as with the checkpoint system
the simple code will force the designer to be careful and i have include a grid in the editor to help creating proper dimensioned levels.
To conclude this project was made to offer a template to let a level designer create a full with a drag and drop system without having to touch
the scripts.
Features
- Unity events
- shape sprite path
- tweeners
- template oriented
Inspiration from the original
- Checkpoints
- Physics system
- Bouncers
- Lives system
Personnal additions
- Ennemies
- Bonus/Malus system
- Open areas