Colorckpick
A 2D infinite mobile puzzle game
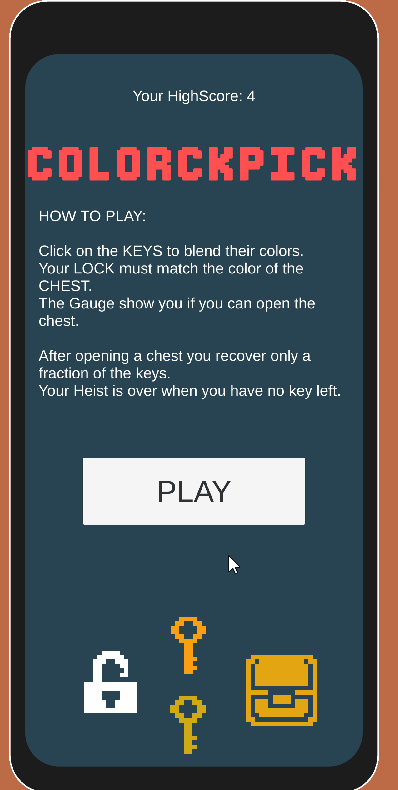
UNITY Color puzzle 2D
This game was done within a one week limit. The goal was to create a small mobile game and to publish it on the Google Store.
The main mechanic is color mixing, the player must match the color of the chest at the top of the screen by clicking on the keys.
Each time a key is clicked its color will add to the mix show by the lock color. The mechanic is inspired by the board game Soviet Kitchen.
Once the lock color is closed enough of the chest one as show by the meter in the top center of the screen ,the player can click on the chest
to earn some points and find a new chest to match.
There is no win condition beside a high score, and the lose condition is reached once there is no key left in the game.
Difficulty management
The difficulty is only managed by the percentage to reach at each level. The player must create a color the closest to the chest one.
At the beginning the goal is 60% and can be up to 90%. If the player mix a color with a matching rate of at least 90%, a bonus is added
to their points.
The simple linear equation of difficulty allows to create a non linear succesful matching. The difference of winning set of keys
between 60% and 70% is greater than between 80% and 90%. This rules is explained in game design books using usually the jump distance
and distance between obstacles in Mario bros. .
The next code shows how the chest color is set by mixing 3 existing key colors.
public void CreateGoalColor()
{
Color newColor = (ObjetSpawner.instance.objectsInGame[Random.
Range(0, ObjetSpawner.instance.objectsInGame.Count - 1)].transform.GetChild(0).
GetComponent().color +
ObjetSpawner.instance.objectsInGame[Random.
Range(0, ObjetSpawner.instance.objectsInGame.Count - 1)].
transform.GetChild(0).GetComponent().color +
ObjetSpawner.instance.objectsInGame[Random.Range
(0, ObjetSpawner.instance.objectsInGame.Count - 1)].transform.GetChild(0).
GetComponent().color) / 3;
goalColor = newColor;
}
The most interesting part of the game is the method to compare colors. We have to use the Unity Color methods to convert RGB to HSV because we want to compare the distance between two points on a circle (two colors on a color wheel). First each Distance is calculated (saturationValue, valueValue and hueValue) by taking the smallest distance value to the limits of 0 and 1. Then we create the current lock color value by taking square root of the sum of squared values (because we are calculating distance on a graph).
public void CalculScore()
{
float valueValue;
float saturationValue;
float hueValue;
float H1, S1, V1, H2, V2, S2;
Color.RGBToHSV(goalColor, out H1, out S1, out V1);
Color.RGBToHSV(currentColor, out H2, out S2, out V2);
// Debug.Log("H" + H1 + "||" + H2 + "|| S" + S1 + "||" + S2 + "|| V" + V1 + "||" + V2);
saturationValue = (Mathf.Min(Mathf.Abs(S2 - S1), 1 - Mathf.Abs(S2 - S1)));
valueValue = (Mathf.Min(Mathf.Abs(V2 - V1), 1 - Mathf.Abs(V2 - V1)));
hueValue = (Mathf.Min(Mathf.Abs(H2 - H1), 1 - Mathf.Abs(H2 - H1)));
matchingRate = Mathf.Round(100 * (1 - Mathf.Sqrt(hueValue * hueValue +
valueValue * valueValue + saturationValue * saturationValue)));
// Debug.Log("hue"+hueValue+"|| saturation:"+ saturationValue + "||
value:" + valueValue + "\n matching:"+ matchingRate);
}
The game was pretty fun to do, especially the trial and error to find the right equation for the color mixing. It allowed me also to discover the Google store process. The dowside of this game is that we dont play with bright color and have to deal with very muted color because of the wide spectrum of grey on the HSV color wheel.
Features
- Unity Device Simulator
- Mobile dev
- Google Store release
- One week project